DataStax Spring Boot Starter for the Java Driver | Datastax
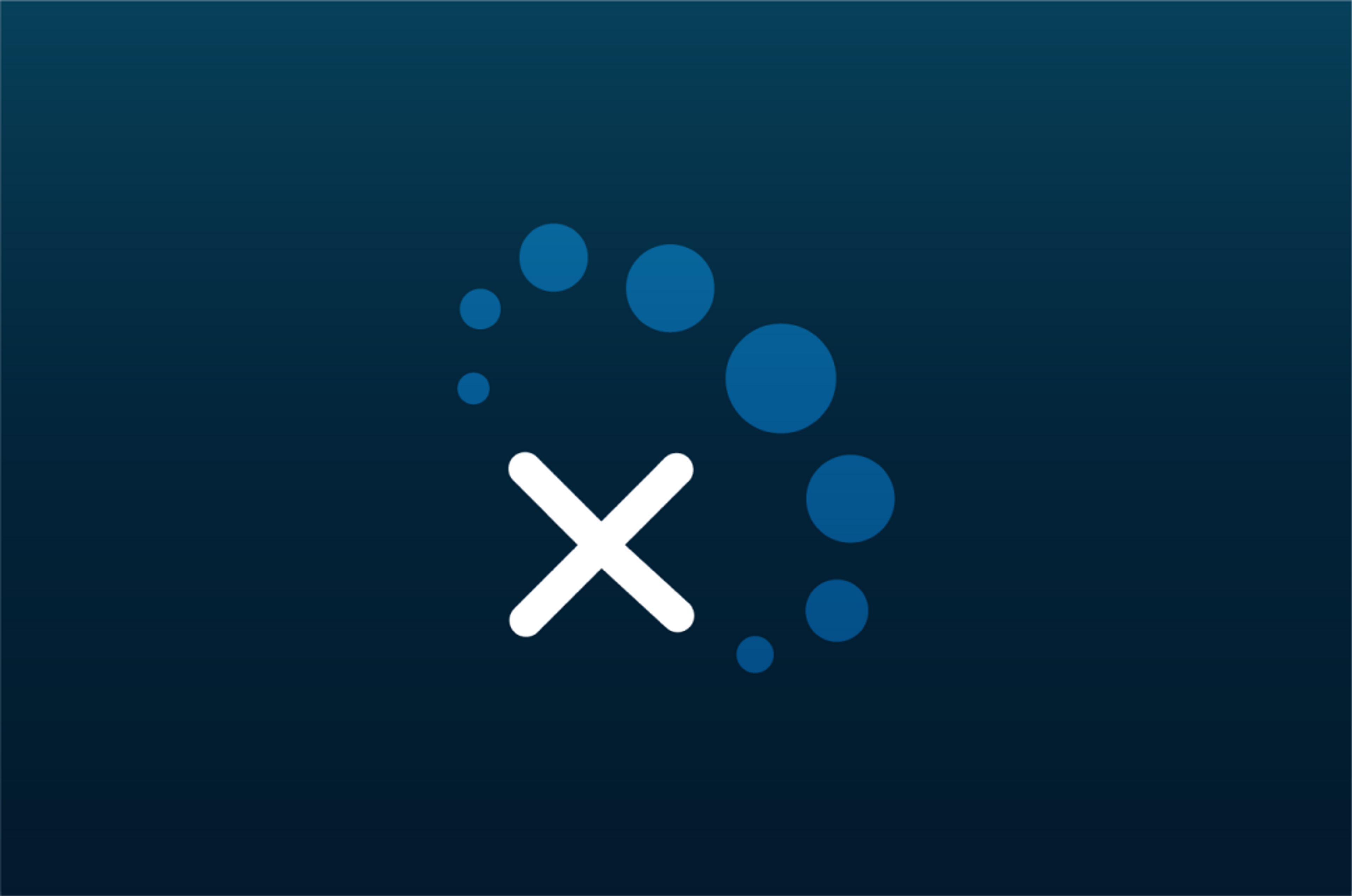
Today we released into DataStax Labs the DataStax Java Driver Spring Boot Starter. This starter streamlines the process of building standalone Spring-based applications that use Apache Cassandra™ or DataStax databases. This is preview software so we have not yet pushed this to Maven Central and instead provide a tarball artifact that contains the starter jar, the starter test jar, README files, the demo described later in this blog post, and an
To download the starter tarball, visit the DataStax Labs downloads page and find the artifact in the box on the right-hand side of the screen.
To install the starter and the test module, run the install_maven.sh script
from the extracted tarball directory. If you hit any issues along the way, post a question on DataStax Community and we’ll help you out. We also have the documentation and the demo on the DataStax Labs Github.
Auto-Configuration
To build Spring applications with Cassandra or DataStax, you’ll need the DataStax Java Driver included and configured in your project, and the DataStax Java Driver Spring Boot Starter makes that easy by providing a familiar way of configuring the driver through Spring properties and profiles. The following is an example of configuring the driver in a Spring application.yml file (you can use application.properties as well if you prefer):
application.yml
datastax-java-driver:
basic.contact-points:
- 10.0.0.1:9042
- 10.0.0.2:9042
basic.load-balancing-policy:
local-datacenter: Cassandra
Driver Access
In the Spring application world, dependency injection is the way to get various Java objects into your application code quickly without having to write all the boilerplate code to construct them. For Cassandra and DataStax applications, the starting point for connecting to the database is the driver: more specifically, it's the driver's CqlSession. The DataStax Java Driver Spring Boot Starter makes it easy to inject a fully configured CqlSession
into your application and start executing queries immediately. An example of using the @Autowired
annotation to do this injection is below, alternatively @Inject
can be used.
@Component
public class MyService {
@Autowired
private CqlSession cqlSession;
public ResultSet execute(String query) {
return cqlSession.execute(query);
}
}
Note that right now the starter uses CqlSession
, support for DseSession
will be coming in the future.
Integration Testing
The Starter even comes with two flavors of embedded integration test options: Docker and CCM. These allow you to write integration tests that will spin up a Cassandra instance for you, run your tests against it, and shut it down as part of your regular application build/CI process. For Docker, the Testcontainers project is leveraged to start an instance of Cassandra. For CCM, we leverage test infrastructure modules that are published alongside the DataStax Java Driver.
Demo
But wait, there’s more! We included a demo project in the labs tarball and also have it hosted at the DataStax Labs Github.
This demo Spring Boot Application shows how to:
- Include the DataStax Spring Starter in an application. See the dependency and the MainApplication class annotated with
@SpringBootApplication
. The driver’sCqlSession
is automatically injected into the spring components. - Leverage the driver’s Object Mapper to map Java objects to Cassandra or DataStax tables. Within the mapper directory, see the
Product entity
, theProductDao
, and theInventoryMapper
. - Expose a REST API to give easy data access. Within the controller directory, see
ProductController
and note that the REST API is usingProductDto
from the api directory. When designing REST APIs this separation is important because it allows you to evolve the REST API independently from the Persistence layer. The DTO to Entity mapping is happening in theProductService
in the service directory. - Build integration tests that use the DataStax Spring Starter. See the separate test dependency and ProductServiceIT. This uses the
@SpringBootTest
annotation that automatically scans all components defined by the class annotated with@SpringBootApplication
and starts the spring application.
Before running the demo, make sure that you have an instance of a Cassandra or DataStax database started and that you are pointing to it in the application.yml. Also, double-check that your local-datacenter name is defined correctly in that yml file.
Once the demo app is running, you can interact with the REST API via curl commands:
# create an entity
curl -d '{"id":1, "description":"i was created by curl"}' -H "Content-Type: application/json" -X POST http://localhost:8080/product/
# retrieve
curl -X GET http://localhost:8080/product/1
# delete
curl -X DELETE http://localhost:8080/product/1
# retrieve - NOT_FOUND
curl -X GET http://localhost:8080/product/1
Try it out!
We hope that the DataStax Java Driver Spring Boot Starter helps you build your Spring-based applications faster with Cassandra and DataStax. Let us know what you think at DataStax Community. Happy coding!