Technology•April 10, 2024
Making Astra DB easier for MongoDB developers
Great news for developers with MongoDB experience and apps! DataStax has updated the Astra DB Data API to be compatible with the MongoDB API.
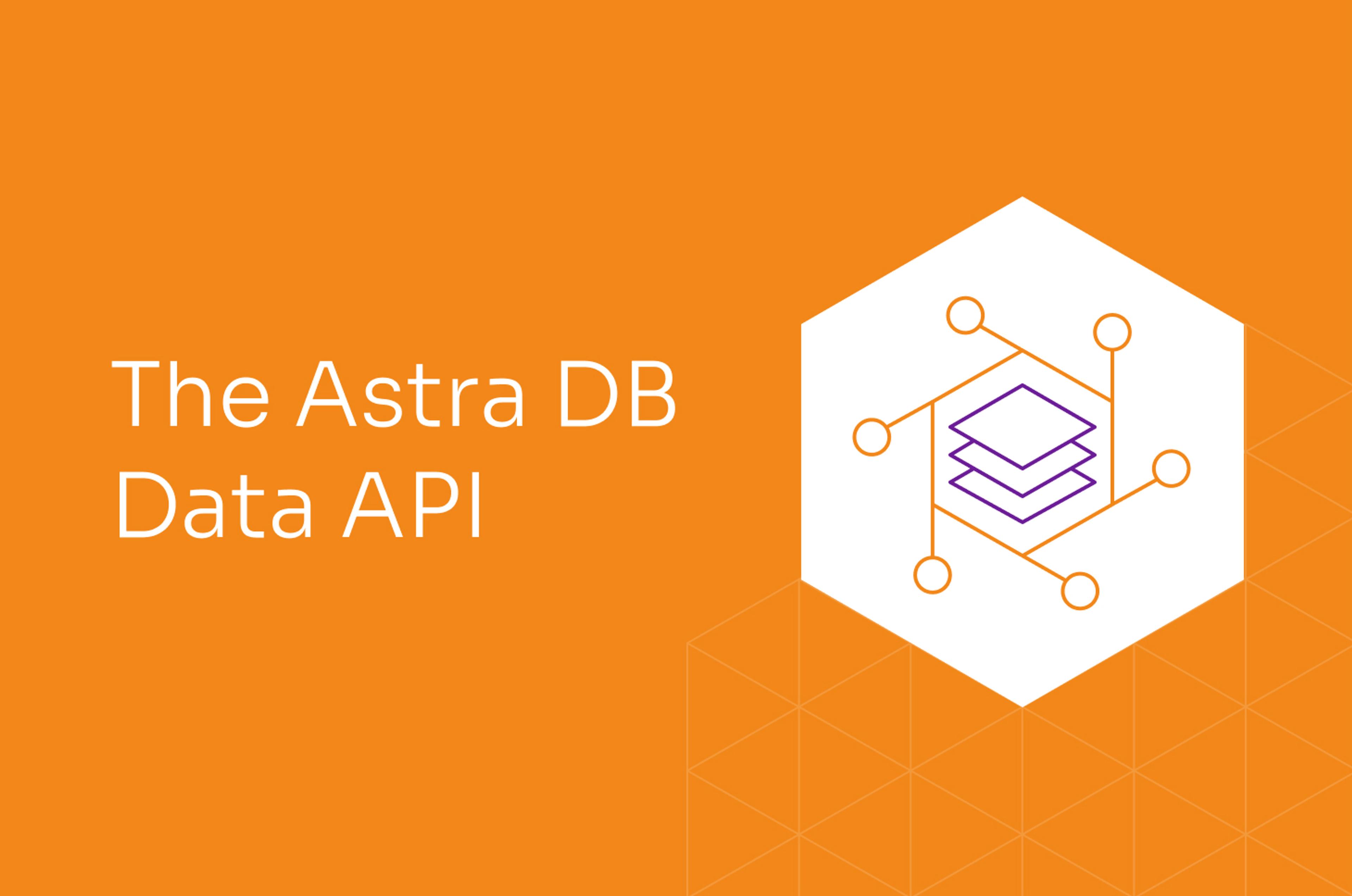
const { MongoClient } = require("mongodb"); // Replace the uri string with your connection string. const uri = "<connection string uri>"; const client = new MongoClient(uri); async function run() { try { const database = client.db('sample_mflix'); const movies = database.collection('movies'); // Query for a movie that has the title 'Back to the Future' const query = { title: 'Back to the Future' }; const movie = await movies.findOne(query); console.log(movie); } finally { // Ensures that the client will close when you finish/error await client.close(); } } run().catch(console.dir);
import { DataAPIClient } from '@datastax/astra-db-ts'; const token = "<Astra DB Token>"; const endpoint = "<Data API Endpoint URL>"; const client = new DataAPIClient(token); async function run() { try { const database = client.db(endpoint); const movies = database.collection('movies'); // Query for a movie that has the title 'Back to the Future' const query = { title: 'Back to the Future' }; const movie = await movies.findOne(query); console.log(movie); } finally { // Ensures that the client will close when you finish/error await client.close(); } } run().catch(console.dir);
{ _id: '51a5d5df-ee2d-4534-a5d5-dfee2de53445', title: 'Back to the Future', year: 1985, genre: 'Comedy', description: 'Marty McFly, a 17-year-old high school student, is accidentally sent 30 years into the past in a time-traveling DeLorean invented by his close friend, the maverick scientist Doc Brown.', '$vector': [ -0.011504995, -0.017071927, -0.014845154, -0.030772243, -0.011385478, -0.011259672, -0.018380314, -0.014467735, -0.023198698, 0.017864507, 0.015599992, 0.019600635, -0.004846691, 0.007542093, 0.034319982, -0.019059667, 0.018342571, -0.011341446, 0.0115364455, -0.013486445, -0.011523865, -0.014706767, 0.0027378616, -0.018770313, 0.005375078, 0.0015191121, 0.008951125, -0.0181287, 0.03421934, -0.021210957, 0.025576439, -0.0006844654, -0.012932897, -0.02441902, -0.030017404, -0.019172894, -0.0018399184, -0.021953216, 0.0131970905, -0.0049379007, 0.017084507, 0.011794349, -0.0043812073, 0.012467413, -0.0024956842, 0.00024532247, 0.0118069295, -0.024620311, 0.0012879429, 0.010762736, 0.018770313, 0.031225147, -0.0123478975, -0.02679676, -0.0026922568, -0.014442573, 0.0004992941, 0.018858377, -0.0139141865, -0.00944806, -0.0076867705, 0.004488143, -0.008309512, -0.0027189907, -0.007661609, 0.013687735, -0.0004222377, -0.0023777408, 0.008542254, 0.022330634, 0.02991676, 0.006950803, 0.03587998, 0.008762415, 0.0030665307, -0.00473032, -0.00022605836, 0.016681926, -0.023198698, 0.014090315, 0.023148376, -0.026746439, -0.004708304, 0.016178701, 0.030948373, -0.005453707, -0.0061141904, 0.026343858, -0.006321771, 0.0025522972, -0.02284644, 0.022204828, 0.0067683836, 0.014241283, -0.005198949, 0.023601279, 0.0048309653, 0.009743704, 0.011184188, -0.01814128, ... 1436 more items ] }
const similarMovies = await movies .find( {}, { // Provide embedding vector based on the prompt. vector: await embedding("Criminals and detectives"), // Limit to three top results. limit: 3, // Do not include vectors in the output. projection: { $vector: 0 }, } ) .toArray(); console.log(similarMovies); // Function that generates embeddings, for reference. async function embedding(prompt) { const response = await fetch("https://api.openai.com/v1/embeddings", { method: "POST", headers: { "Content-Type": "application/json", Authorization: `Bearer ${OPENAI_API_KEY}`, }, body: JSON.stringify({ input: prompt, model: "text-embedding-ada-002", }), }); const result = await response.json(); return result.data[0].embedding; }
[ { _id: '4f80198d-5456-4ea6-8019-8d54567ea6c6', title: 'The Criminal Hypnotist', year: 1909, genre: 'Drama', description: 'The Criminal Hypnotist is a 1909 American silent short drama film directed by D. W. Griffith.' }, { _id: 'f3ddb56a-2d02-4303-9db5-6a2d02b303bd', title: 'The Amateur Detective', year: 1914, genre: 'Comedy', description: 'The Amateur Detective is a 1914 American silent short comedy directed by Carroll Fleming for the Thanhouser Film Corporation. The film stars Carey L. Hastings, Ernest C. Warde and Muriel Ostriche.' }, { _id: 'deb0e739-9d6f-489c-b0e7-399d6fd89c9f', title: 'Hemlock Hoax, the Detective', year: 1910, genre: 'Comedy', description: 'Hemlock Hoax, the Detective is an American short comedy film produced and distributed in 1910 by the Lubin Manufacturing Company. The silent film features a detective named Hemlock Hoax who tries to solve a murder, which unbeknownst to him is a practical joke being played on him by two young boys. It was one of many shorts designed to derive its humor from a sleuth whose name was similar to Sherlock Holmes.' } ]