Pulling Real-Time Ethereum Transactions with Web3.js
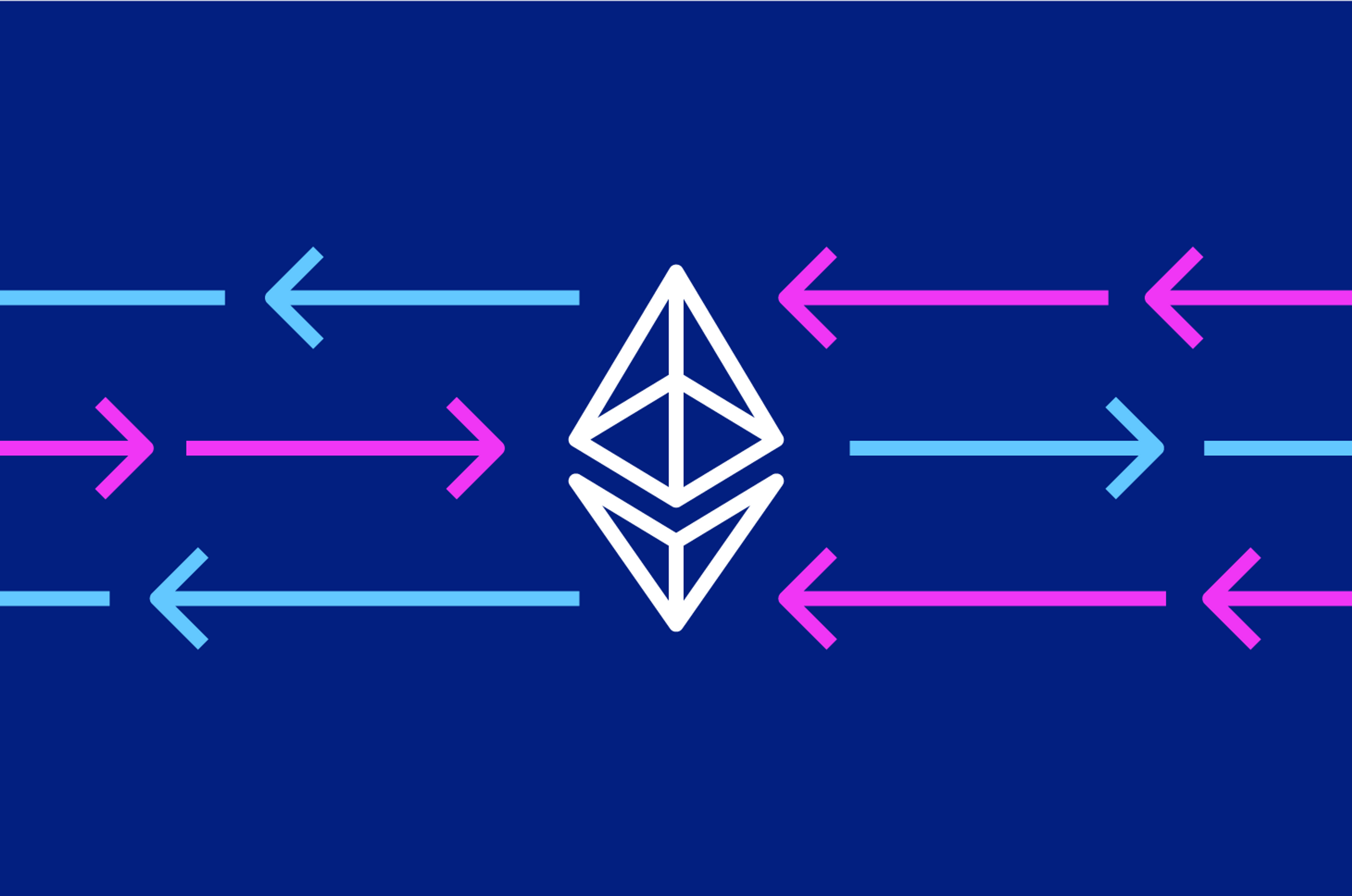
This is the second post in our brand new Astra DB Crypto Blog Series. This series covers everything you need to know about Web3. If you want an introduction to NFTs, and a walkthrough of how you can load them into DataStax Astra DB, have a look at Part 1.
This week on the DataStax crypto series we're covering RPC Node basics, how to connect to a RPC node, and viewing real-time transactions. Ethereum uses JSON-RPC (Remote Procedure Protocol) API to ensure uniform methods for applications interacting with the Node. Developers can utilize a variety of languages to interact with a RPC Node (Go, C#, Java). This post will also give a brief background of what Web3.js is and how it can be used in relation to the Ethereum blockchain and in gathering Ethereum transaction data.
Getting Familiar with Web3.js
Web3.js is a collection of libraries that give the user access to local or remote Ethereum nodes using HTTP, IPC, or WebSocket. Developing blockchain applications on the Ethereum blockchain comes with a couple of different aspects to keep in mind: making sure your content interacts with the blockchain and developing smart contracts that get deployed accordingly. Web3.js allows you to accomplish the former aspect by developing clients that interact directly with the Ethereum blockchain. Among the various functions that Web3.js offers, like sending Ether from one account to another or creating and editing smart contracts, it generally helps to streamline the process of interacting with the Ethereum blockchain. Web3 API will help you pull Ethereum transaction data, any transaction hash, account address, or smart contract that you need. The functionality of Web3.js is vast and invaluable within the Web3 space, as it allows you to accomplish a great deal inside the Ethereum blockchain.
Now that you have some background on what Web3.js is, let’s get started with how you can use Web3.js to pull real-time Ethereum transactions from the blockchain.
Ethereum Node Basics
In short, nodes (in the cryptocurrency and blockchain world) are computers running Ethereum client software. Nodes connect with each other to send information back and forth, validating transactions and storing data about the current state of the blockchain. In essence, nodes are what makes up the entirety of the blockchain network and are the only way to access technology like the Ethereum blockchain. When pulling real-time Ethereum transactions, you’re writing data on the blockchain and updating its current state. You can get a greater understanding of what this process is like by utilizing web3.js and Ethereum nodes.
To start getting information from the Ethereum blockchain, you will first have to set up a node. Follow the steps below to get started:
- An Ethereum Node is a server running the Ethereum software that verifies existing blocks and stays in sync when new blocks are mined.
- You can run your own Ethereum Node or purchase a “node-as-a-service” from companies like Ankr ($), Infura ($$$) or Alchemy ($).
- The most popular setup for running your own Ethereum Node is Geth running on AWS. You can view other popular setups here.
- You can make GraphQL, Websocket or HTTP API calls to RPC nodes to receive blockchain data.
- Client libraries like Web3.js make it super easy to make API calls to RPC node.
Pulling transaction data
Once you have your Node set up, it's simple to pull Ethereum data using Web3.js. You can connect to your node with this Web3.js collection of libraries.
Step 1. Set up a basic Node.js project.
mkdir w3 cd w3 yarn init touch app.js
Step 2. Install Web3.js.
yarn add web3
Step 3. Add the following to the top of app.js.
const Web3 = require('web3');
const RPC_ENDPOINT = “REPLACE_ME”;
const web3 = new Web3(RPC_ENDPOINT); #replace RPC_ENDPOINT with the endpoint you spun up in step 1.
Step 4. Listen for new blocks using web3.js. The second a new block is mined, the last block number will be logged.
const subscription = web3.eth.subscribe(
"newBlockHeaders",
(err, result) => {
const { number } = result;
console.log(number)
});
Step 5. Now that you have the latest block number, we can make a getBlock()
call to pull data on the latest transactions.
web3.eth.getBlock('123').then(blockResults => console.log(blockResults))
The getBlock()
call will return all the transactions processed in the block, for block #123, here are the results.
{ difficulty: '18118731572', extraData: '0x476574682f4c5649562f76312e302e302f6c696e75782f676f312e342e32', gasLimit: 5000, gasUsed: 0, hash: '0x37cb73b97d28b4c6530c925d669e4b0e07f16e4ff41f45d10d44f4c166d650e5', logsBloom: '0x00000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000', miner: '0xbb7B8287f3F0a933474a79eAe42CBCa977791171', mixHash: '0x2e4b92a11b1bac2a311f6a47006442bf1dc689e76c9c1fee90da56ff6f2df7a7', nonce: '0x18c851620e8d6cb6', number: 123, parentHash: '0x701bc7632e80976d1a2c408ffa58e4f11aa3ed3c5a030d1125930a9d944e4343', receiptsRoot: '0x56e81f171bcc55a6ff8345e692c0f86e5b48e01b996cadc001622fb5e363b421', sha3Uncles: '0x1dcc4de8dec75d7aab85b567b6ccd41ad312451b948a7413f0a142fd40d49347', size: 542, stateRoot: '0x2d1e6407139174d74e9485ce0b9f80d31f6ec55f447708796d2582e3ffbdbb85', timestamp: 1438270492, totalDifficulty: '2181259381686', transactions: [], transactionsRoot: '0x56e81f171bcc55a6ff8345e692c0f86e5b48e01b996cadc001622fb5e363b421', uncles: [] }
Final notes
This post presents a straightforward approach to get you started reading blocks within minutes. It's the backbone of any application built on the blockchain.
Now that you have all the data, the building options are endless. Continue following along with this series for more fundamental knowledge on developing with the blockchain! The world of decentralized finance requires easily available data in order to keep up with the fast-moved, instantaneous user experience the blockchain offers. That’s why DataStax offers Astra DB: a true peer-to-peer, 24/7 database for all of your finance and cryptocurrency needs. Whether you’re looking for real-time trade analytics, charting Ethereum transactions, or keeping record of smart contracts, DataStax has you covered. Have full visibility of Ethereum transactions data across all of your users and make your finances fit for Web3 and Ethereum transactions. Find out more about Astra DB and how it can help you with your cryptocurrency finances.
If you have any questions or need help with any of the instructions above, ping us at hello@datastax.com.